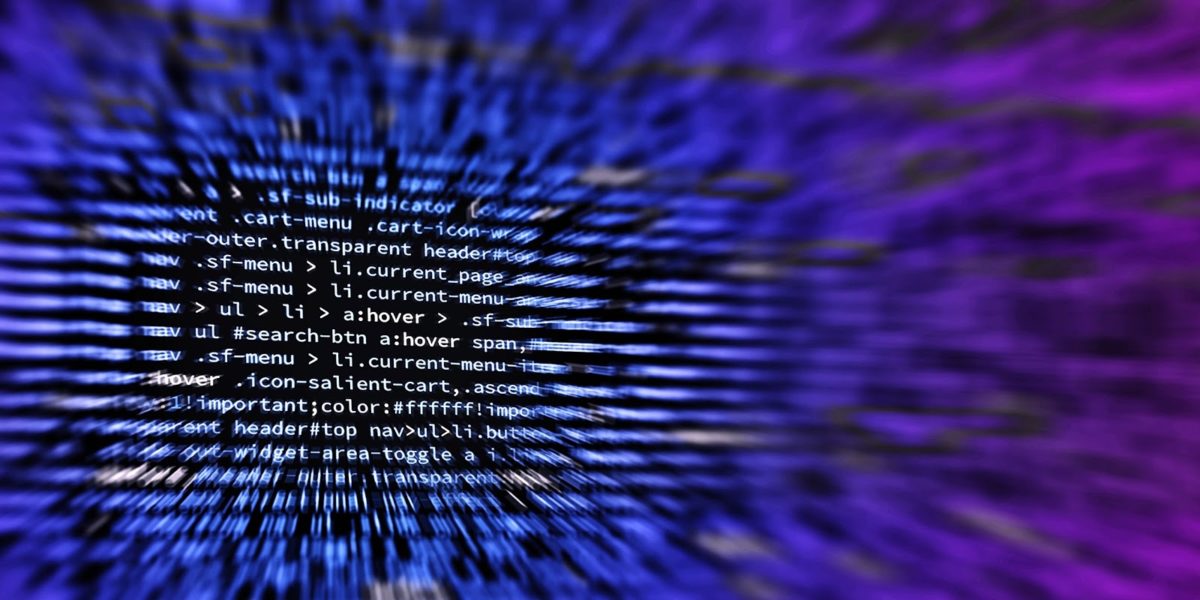
C/C# Common Programming Statement Keywords
For those developers that are interested in starting to learn new Common Programming Statement Keywords, I will feature various tutorials on how to use basic code within each environment. Feel free to leave comments for clarification or request to expand on a new topic.
**The basic statements featured in this blog will cover syntax shared by the C/C# language. I use Microsoft Visual Studio 2010 to compile my code.
If/Else Statement
Example #1: Basic Statement
//This line of code checks to see if the condition stated is true. If true, the statement1 is executed.
//If the condition is false, the statement 2 is executed
if (condition)
statement1;
else
statement2;
Example #2: Multiple Conditions
//Notice how brackets {} are require if more that one statement is used.
//If more that one condition is required, ‘else if()’ can test additional conditions.
if (condition1)
{
statement1;
statement2;
}
else if (condition2)
statement3;
else
statement4;
For Statement
Example #1: Basic Statement
//for statements create loops. for(initialize variable, set condition, increment counter){}
for(int i= 0; i < 5; i++)
{
x = i;
}
Example #2: Nested Loops
//Nested loops are loops inside of other loops. The inner loop executes fully before the outer loop
//is incremented.
for(int i= 0; i < 5; i++)
{
for(int j= 0; j < 4; j++)
{
x = i*j;
}
}